Continuing the series of building a multiplayer poker game, some progress has been made on the game. Development is coming along great using Phaser Editor v3!
A Bit More Technical Setup
I’m using several technologies, as mentioned in the first article of this series, and as I’m moving forward, I’m continuing to make adjustments to my development environment.
Since the game will perform as both a single-player and multiplayer game, I’m working on the single-player aspect first.
The game will be split into two primary projects: one for the client-side, and the other for the server-side.
Both will use common logic for running the game, and to avoid having to duplicate code across both projects. I made a secondary project, which is acts as a “common” repo for sharing code that will be used by both projects.
Since the common folder is outside of the client project root folder, I made come adjustments to VSCode to allow it to “see” this external folder and add it to the workspace.
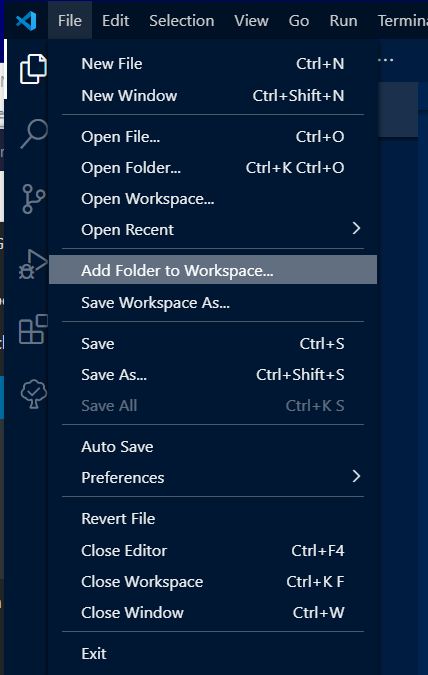
The common folder is in the same folder as the client project (and server project). In order for Webpack to add the common files and folders to the build process, I made some adjustments to the webpack.config.js
file. The alias
inside of the resolve
also makes the import
statements look cleaner in VSCode.
module: {
...
rules: [
...
{
include: [
...
path.resolve(__dirname, '../common/src'),
],
...
}
]
};
...
resolve: {
...
alias: {
common: path.resolve(__dirname, '../common/'),
}
};
Learning Poker
I’m learning the rules of poker as I build this game, and the journey has been quite interesting. π
The order in which the players take their turns was fairly straightforward. Play starts with player left of the dealer, small blind (SB). The player left of the SB is the big blind (BB). Play goes clockwise around the poker table.
The object of poker is to win all the money in the pot. You do this by having the best poker hand at the end of the game. Your hand must be built from any combination of two cards that you’ll eventually be dealt (the “hole” cards), plus the five community cards that will eventually be dealt. As players make their turns, they can place bets by adding money to the pot. The player who wins the game (by having the best poker hand) at the end of the game wins all the money in the pot.
The game is split up into rounds and each round doesn’t end until every player has contributed an equal amount of money to the pot.
Note: I’ll be using 1/2 no-limit (NL) rules.
To start the game off, the SB posts a forced bet bet of 1bb, and the BB posts another forced bet of 2bb, twice the SB’s.
After both blinds make their bets, each player is dealt two face-down cards. (You can see your own cards, of course π)
When it’s the next player’s turn, if they wish to stay in the game, they must “call” by matching the BB’s bet of 2bb.
When play returns the the SB, they need only post 1bb, since they posted the initial 1bb star started the game. After this, all players still in the game (who haven’t quit, or folded) have posted an equal amount of 2bb.
After all players have placed equal bets the betting round comes to a close, and the first three of five community cards, the flop, are dealt, face-up in the center of the table.
The round begins again with the player nearest the left of the dealer. This would be the SB if They haven’t folded. Each players has the following moves:
- Check: Pass to the next player without making a bet.
- Bet: Increase the current bet by posting additional money into the pot. If a player does this, all other players must either match this bet, fold, or re-raise. More on re-raise later.
- Fold: Forfeit their place in the current game. Their hand is surrendered face-down. Note that they can return to play the next game.
- Call: If a previous raise has been made, the player can call by matching the previous bet.
- All-In: If a player does not have enough money to match the current bet, and they wish to stay in the game, they must go “all in”, betting all their remaining chips. Note that if the player does not win this game, they will lose all their money and be out of the game!
Once the flop round is complete, the fourth community card is dealt, called the turn. Betting begins again with the player left of the dealer. And the round ends once all players have posted equal amounts to the pot.
Note: It is possible to no players to make any bets during a betting round by simple calling each time. But that would make for a slow and boring game, IMO. Maybe that’s the rookie talkin’, haha! π
Once the turn round is complete, the fifth and final community card is dealt, called the river.
(Image owned by Activision.)
Couldn’t resist.
Ahem! Anyway, betting begins again with the player left of the dealer. And the round ends once all players have posted equal amounts to the pot.
After this round ends, the showdown begins!
During the showdown, all players still in the game reveal their hands, and the player with the best hand wins the pot. If you’re curious, you can learn more about the poker hands here.
Re-Raising
Back to the concept of re-raising. This is when a player raises the bet, then another player raises again after them. Depending on the type of poker being played, the rules for determining the minimum (and maximum) amounts you can raise will vary. Since I’m coding a game using the rules by No-Limit, the maximum you can bet is all your available chips. However, the minimum you can bet can be a little trick to figure out, because it’s simply not just “double what the big blinds bet”.
I’m still working on this part, but I think I’ve got it (if not close π ). Of course, if you’re an expert on calculating the minimum amount for re-raising in No-Limit 1/2 poker, please comment and correct any of my assertions. That would be greatly appreciated. ππΎ
When re-raising against previous raise, the minimum amount must be at least the amount raised by the previous player. Take this example below.
Pot size is 6bb.
Player 1 bets 4bb.
Player 2 raises to 10bb.
What would be the minimum raise for Player 3?
First, let’s have a look at the wording used. Player 2 raised to 10bb. However, Player 2 actually increased the bet amount by 10 – 4 = 6bb. So, the minimum Player 3 will need to raise by is 6bb.
This would be a total raise size of:
10 (previous player’s total raise to) + 6 (minimum raise by) = 16bb.
Back To The Game Dev
As I close this article, here’s a screen shot of the current progress. I’m currently working on the raise (and re-raise!) implementation, as well as a few other functions, such as folding and going all-out.
After that, I’ll move on to the showdown functions and determine the winner among the various conditions.
Thatβs all for now. Iβll continue to keep you updated as I make progress.
Finally, you can sign up using the form below to receive updates to playable builds (or at least interactable builds) as I make noteworthy progress.
Talk to you later,
– C. out.