There is one more type of hand the player can win: getting a Blitz. So we’re going to code that functionality into the game in this article.
If you’re familiar with Blackjack, you might also know what a Charlie is. If not, don’t worry about it. I gotcha. 👊🏿
A Charlie is when a hand has acquired so many cards – usually five, six, or seven – without busting. When a player gets a Charlie, they are paid immediately, just like when getting a Blackjack or a Thunderjack.Also, the player does not play against the dealer. The next player’s turn immediately begins afterwards.
Thunderjack! will adopt a six-card Charlie, and I’m calling it a Blitz. Why? Because Blitz just sounds cooler. 😎
Ha! But no, taking the definition of the word (currently defined by Merrima-Webster, which is being “a fast intensive nonmilitary campaign or attack”.
So, if you’re taking hit after hit after hit, and still haven’t busted, I’d say that’s a pretty “intensive attack” – on attempting to beat the dealer. Would you agree? 😉
Determining if the player has a Blitz can be checked each time the player takes a hit and has not busted. You can modify the the beginTurnPlayerHit
function which was defined in the article that introduced the hit functionality. The updated function is shown below:
beginTurnPlayerHit
GamePlay.prototype._beginTurnPlayerHit = function() {
//get the playerData of the current turn player. we'll be using this as a paramater to the other functions
var turnPlayer = this._getTurnPlayer();
this._dealCardToPlayer(turnPlayer);
this.updateScores();
if (this.didPlayerBust(turnPlayer)) {
this._beginPlayerBust(turnPlayer);
this._setNextTurnPlayer();
} else {
if (this._doesPlayerHaveBlitz(turnPlayer)) {
this._beginPlayerBlitz(turnPlayer);
this._setNextTurnPlayer();
}
}
};
doesPlayerHaveBlitz
GamePlay.prototype._doesPlayerHaveBlitz = function(playerData) {
var playerHand = playerData.getHandData();
var NUM_CARDS_FOR_BLITZ = 6;
return(playerHand.getNumCards() === NUM_CARDS_FOR_BLITZ &&
playerHand.score <= Thunderjack.DesignData.BLACKJACK_POINTS);
};
This function checks that the player’s hand has exactly six cards, and that the score does not exceed 21 (the number of Blackjack points).
Note: Note: You can change NUM_CARDS_FOR_BLITZ
to a different value if you like. If you do, make sure your player hand user interface can handle that number of cards. With the current setup of the player_prefab canvas defined in Phaser Editor.
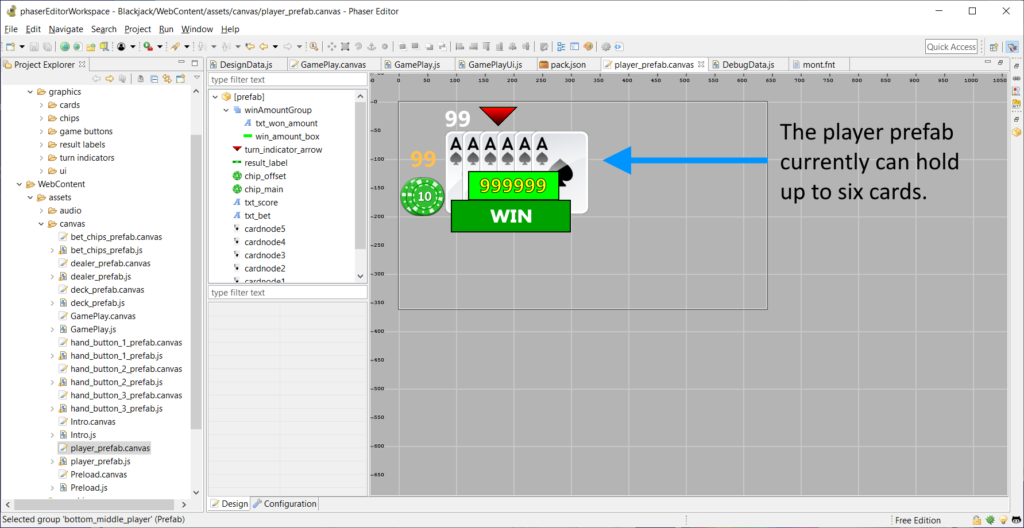
beginPlayerBlitz
GamePlay.prototype._beginPlayerBlitz = function(playerData) {
playerData.hasBlitz = true;
//player is paid immediately here as well
};
A player who gets a Blitz does not play against the dealer. To start this off, the hasBlitz
property is set to true
in the beginPlayerBlitz
function. Next, we’ll need this to update the isPlayingVsDealer
function.
The isPlayingVsDealer
function determines if a player is playing against the dealer. Our last implementation of the function looked like this:
function isPlayingVsDealer(handData) {
return(
!(playerHandData.hasBlackjack ||
playerHandData.isPush ||
playerHandData.isBust ||
playerHandData.hasThunderjack));
}
We’ll update that so that to include players who have not Blitzed are eligible to play against the dealer:
isPlayingVsDealer
function isPlayingVsDealer(handData) {
return(
!(playerHandData.hasBlackjack ||
playerHandData.isPush ||
playerHandData.isBust ||
playerHandData.hasBlitz ||
playerHandData.hasThunderjack));
}
Finally, in the beginTurnPlayerHit
function, after the player has Blitzed, the next player’s turn begins, with a call to the setNextTurnPlayer
.
That does it for adding Blitz functionality in the game! This function required mostly a few modifications to the existing code.
Thanks for reading this article. We’ll talk to you next time. Take care.
– C. out.