Hey.
When I’m building a game, I prefer to have a visual editor when placing as many objects as possible. This includes user interface such as HUDs and text.
So, I’m adding text to my Tiled map to have Phaser’s Tilemap
object load it for display. I’m using Phaser.BitmapText
, but Tiled uses fonts installed on your system, so showing the text won’t display as easily as, say, tileset tiles.
To add text to a Tiled layer, you need to first create an Object layer, then you can add the text object to it. The text properties of a text object will look something like this:
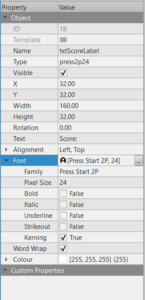
When the map is exported to a format that Phaser can use – I’m using JSON – the format of the object looks something like:
{
"height":32,
"id":18,
"name":"txtScoreLabel",
"rotation":0,
"text": {
"color":"#ffffff",
"fontfamily":"Press Start 2P",
"pixelsize":24,
"text":"Score:",
"wrap":true
},
"type":"press2p24",
"visible":true,
"width":160,
"x":32,
"y":32
}
On the Phaser side, I’ve loaded the JSON map with this:
loader.tilemap('brickout', 'media/graphics/maps/brickout.json', null, Phaser.Tilemap.TILED_JSON);
And created a bitmap font using Littera. It’s loaded into Phaser with this:
loader.bitmapFont('press2p24', 'press2p24.png', 'press2p24.fnt');
Looking back at the JSON format above, you’ll see that the type
property has the value of press2p24
. The type property doesn’t appear to be used by Tiled for anything. On the Tiled’s JSON Map Format page, it states, “String assigned to type field in editor”. So I decided to use the type
property as the key that Phaser uses when defining BitmapText objects.
Also, in the JSON format, some other key properties include x
, y
, text.pixelsize
, and text.text
.
I first tried to get the text data by creating a Phaser.TilemapLayer
from the layer (named ‘text’) like this:
map.createLayer('text');
This is a layer I used exclusively for text objects in Tiled. So, I’d then iterate through all the elements in the ‘objects’ property of the Phaser.TilemapLayer
and create my BitmapText objects like this:
game.add.bitmapText(object[i].x, object[i].y, object[i].type, object[i].text.text, object[i].text.pixelSize);
However, the text was not showing up in the game. It seems all the properties were loaded into Phaser except for the text
sub-object. The object[i].text
sub-object wasn’t being loaded into Phaser. You can see in this screenshot below, when I inspect the Tilemap object look at the objects property, the first element (0) of the text layer does not have a text
sub-object in it.
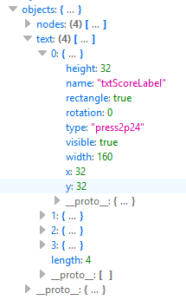
So, Phaser was not loading in that data. When I inspected the code on GitHub (I tracked it down to the src/tilemap/TilemapParser.js
.), the text object was eventually being loaded as a Tiled rectangle object, and there is no code for loading the text sub-object.
But, the raw JSON data that Phaser loads does include all the information needed, including the text
sub-object, as shown:
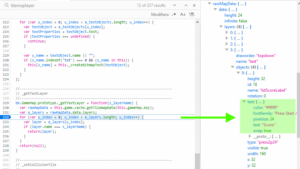
I was able to obtain the raw data with this code:
var rawMapData = game.cache.getTilemapData(tileMap.key);
Then I could get the text
objects layer from with rawMapData.data.layers
. And from there, the text
sub-object to retrieve the text
and pixelSize
properties.
It seems like quite a bit of work, but not really. Going through the learning process was longer than the solution, actually. (: I just needed a way to specify the data in a visual editor and have it supported in code. I’d prefer not hard-code positions and other visual aspects that should be defined in a visual editor.
And If you’re wondering the font is Press Start 2P. (:
– C. out.