Hey there,
The mini-series about writing code to match poker hands continues on! This article covers how to match a three of a kind.
You can also find links to the previously covered poker hands in the list below. The list also includes the ones we’ve yet to cover.
- Pair (jacks or higher only)
- Two pair
- Three of a kind
- Straight
- Flush
- Full house
- Four of a kind
- Straight flush (a non-ace-high straight flush)
- Royal flush (an ace-high straight flush)
Setup
The setup was also explained in the first article of the mini-series, you can check that out here. There, you’ll also a detailed description of the data structures used. But here is a quick rundown of the data structures used in the code:
Card values
CardValues = {
two: 2,
three: 3,
four: 4,
five: 5,
six: 6,
seven: 7,
eight: 8,
nine: 9,
ten: 10,
jack: 11,
queen: 12,
king: 13,
ace: 14,
wild: 100
};
Card suits
CardSuits = {
hearts: 1,
diamonds: 2
clubs: 3,
spades: 4
};
Card
Card = function(value, suit) {
this.value = value;
this.suit = suit;
};
Hand
And a hand is an array of Card
objects:
var hand = [
new Card([card value 1], [card suit 1]),
new Card([card value 2], [card suit 2]),
...
new Card([card value 5], [card suit 5])
];
Writing The Code To Identify A Three of a Kind
A three pair is similar to a pair, except you’re looking for three cards of the same value (rank) instead of two.
As you’ve done before, first you sort the cards by their rank, in descending order. We previously defined a “utilities” file, named CardMatchUtils.js
, and added a function to it called, CardMatchUtils.SortCardsByDescendingValue
, to help with that. You’ll be sorting the cards by descending value for all of the poker hands, so it’s very handy to have this reusable function.
You’ll also be using the “wild card”, which is a card that can assume the rank of any card. The image of the following hand would also qualify as a three of a kind:
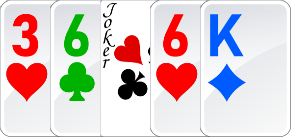
The main function for the pseudocode for matching a three of a kind looks like:
ThreeOfAKind = FUNCTION(hand) {
SORT the hand by descending card value and store in a variable called sortedHand
IF CardsHaveSameValue(sortedHand, 3)
//we have a three of a kind!
RETURN TRUE
//no three of a kind in this hand
RETURN FALSE
}
As you can see, this function is very short and sweet, because of the CardsHaveSameValue
function, which we previously wrote as the CardMatchUtils.DoCardsHaveSameValue
function. If you haven’t seen CardMatchUtils.DoCardsHaveSameValue
yet, that’s where the brunt of the work is done. This function determines if any cards in the hand have the same rank, specifying the number of cards we want to match, in this case, three.
Now time for a little fun 🙂
Below you’ll find a “hand” of five cards. You can set the suit and value of each card. It will then tell you if the cards you selected match any of the poker hands you’ve looked at so far:
- Pair (Jacks or higher)
- Two pair
- Three of a kind
Each article in this series will introduce the next poker hand that you can explore. Give it a try!
If you have any questions about the above interaction, please let me know at cartrell@gameplaycoder.com.
Finally, if you’d like to hire me to make an actual card game for you, please e-mail me at cartrell@gameplaycoder.com , or you may use the contact form here.
That’s it for now. Thanks, and stay tuned for more as we add more poker hands!
– C. out.
The card hand generator was a lot of fun. I of course attempted impossible hands like having two aces of the same suite haha.
Looking forward to seeing how this progresses!
Yeah, you can select the same card multiple times. I noticed that while I was building it, and thought about handling it, but decided not, because it’s not needed to illustrate the lessons in these posts. Of course, you wouldn’t want to be able to do that in a real game though! (:
Thanks, Shin.
– C. out.