When you have two poker hands and both are a three of a kind, this article will show you how to compare those hands and determine which one wins.
In a previous article for poker hands, you saw how to compare a hand to determine if a hand was three of a kind. A three of a kind is a hand three of the cards are the same value, and the remaining two cards are of different, non-pair values. Here’s an example of a three of a kind:
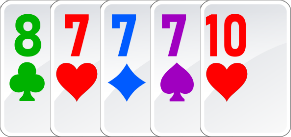
When determining the winner between two hands that are both three of a kind, the process is very similar to the pair’s, except we’re dealing with a trio instead fo a pair:
- Compare the values of the trios.
- If those values are equal, compare the values of other two cards (the “kickers“), starting with the highest one.
- Continue comparing kickers until a kicker from one hand is higher than the other’s.
- If no kicker breaks the tie, and all cards have been compared, then both hands are equal.
Some examples should help with explaining the concepts.
Consider these two hands:
Note: Just like with pairs being first sorted by the pairs,, the cards are sorted first the trio, then by the kickers in descending order of value. The hands above are already sorted this way.
First, compare the value of the trios. Hand 1 has trio of 8s, and hand 2 has a trio of 9s. So hand 2 wins this contest.
Let’s try another. Let’s say the values of the trios of both hands are the same as shown here:
Both hands have the same trio value of three. So you compare the kickers.
The values of first the kicker of each hand is a seven, so we’re tied. Let’s compare the second and final kicker.
You can see that 6 > 4, so the first hand has the higher three of a kind.
And we can’t forget about our crazy wild cat wild cards! 😼 They always seem to throw a 🐵 🔧 into our plans. But we’re going to consider them as well.
Have a look:
In hand 1, we have a trio value of kings, and the a wild card is one of the kickers. Hand 2 also has a trio value of kings (the wild card substituting as a king). Since both hands have the same trio value (king), let’s compare the kickers.
Remember that a wild card can assume any value, and in our case, we want the wild card to assume the highest possible value (while still satisfying the hand and form the highest possible hand: see the note below). In the case of hand 1, the wild card assumes the value of a ace. If you compare that to hand 2’s first kicker, an ace beats a 10. So hand 1 is the winner here.
Note: In hand 1 above, you could’ve also formed the three of a kind by substituting the wild for a king like this:
However, if you use as many non-wild cards as possible to form your three of a kind, when using wild cards won’t increase the trio value, you can save any remaining wilds for as kickers. It will always be substituted as an ace, thus giving you the highest possible hand. When comparing hands, the code introduced in this article will help with that.
Setup
Note: The first stages of the setup can be found on the introduction page to comparing poker hands, here.
There aren’t any other specific preparations we need before we begin.
Writing The Code For Comparing Threes Of Kinds
When two hands are both a pair of jacks or better, the GetWinningHand
function will eventually call a function that compares two hands of said poker hand.
In this case, GetWinningHand
function will eventually call FindWinnerJacksOrBetter
to compare the hands. Just like FindWinnerHighCard
from the last article, FindWinnerJacksOrBetter
accepts two parameters, the hand for both players, and it’ll return the ID of the player who has the winning hand. If both hands are equal, it’ll return 0
(which we’ll use to mean a draw). The pseudocode below starts by comparing the values of the pairs first, then comparing the kickers if necessary.
FindWinnerThreeKind
FindWinnerThreeKind = function(hand1, hand2) {
sortedHand1 = CardMatchUtils.SortCardsByDescendingValue(hand1);
SET hand1Info to an empty object { }
CardMatchUtils.DoCardsHaveSameValue(hand1, 3, hand1Info);
sortedHand2 = CardMatchUtils.SortCardsByDescendingValue(hand2);
SET hand2Info to an empty object { }
CardMatchUtils.DoCardsHaveSameValue(hand2, 3, hand2Info);
SET winningIndex = CompareTrios(hand1, hand1Info, hand2, hand2Info);
IF (winningIndex NOT EQUAL TO -1)
RETURN winningIndex (one hand's trio outranks the other hand's, and no further checking is necessary)
END
(both hands have the same ranking trio, now compare the kickers of both hands)
SET winningIndex = CompareKickers(hand1, hand1Info, hand2, hand2Info);
RETURN winningIndex;
}
Comparing the Trios
First the code above sets the hands up for comparing their trios. It then sort the cards in descending card value. Next, it uses the DoCardsHaveSameValue
function to get the three cards in the hand that make the trio, as well as determine the card value of the trio.
CompareTrios
CompareTrios = function(hand1, hand1Info, hand2, hand2Info) {
SET hand1WorkingTrioValue = hand1Info.cardValues[0]
IF (CardMatchUtils.CountCardsByValue(hand1, WILD_VALUE) GREATER OR EQUALS 3)
hand1WorkingPairValue = ACE_VALUE
SET hand2WorkingTrioValue = hand2Info.cardValues[0]
IF (CardMatchUtils.CountCardsByValue(hand2, WILD_VALUE) GREATER OR EQUALS 3)
hand2WorkingTrioValue = ACE_VALUE
IF (hand1WorkingTrioValue > hand2WorkingTrioValue)
RETURN 0 (hand 1 has the higher ranking trio)
ELSE IF (hand1WorkingTrioValue < hand2WorkingTrioValue)
RETURN 1 (hand 2 has the higher ranking trio)
END
RETURN -1 (both hands' trios have the same value)
}
This function is very similar to the ComparePairs
function in our recent article for comparing pairs of jacks or better, except instead of dealing with two cards, we’re dealing with three. If you’d like details on the workings of that function, please check here.
Comparing the Kickers
If the trios of both hands have the same rank, then you’ll need to compare the other two cards, the kickers.
Like you did when comparing the kickers with previous poker hands, when comparing the kickers, you first remove the trios from both hands so you’re left with the remaining kickers. You can use the CardMatchUtils.BuildHandExcludingValues
function to remove the trio.
You can use this psuedocode to get the kickers in the hand:
GetKickers
GetKickers = function(hand, info) {
(get the value of the cards that form the trio)
SET trioCardValue = info.cardValues[0]
(count the number of cards in the hand that match the trio card value)
SET numTrioValueCards = CardMatchUtils.CountCardsByValue(hand, trioCardValue)
(if there are at least as many trio value cards as there are non-kicker cards, specify the trio card value to the values of cards to exclude)
SET numNonKickerCards = 3
IF numTrioValueCards >= numNonKickerCards
SET valuesToExclude = trioCardValue
ELSE {
(otherwise, if there are at least as many wild cards as there are non-kicker cards, add the wild card value to the values of cards to exclude)
SET numWildCards = CardMatchUtils.CountCardsByValue(hand, CardValues.WILD)
IF numWildCards >= numNonKickerCards
SET valuesToExclude = CardValues.WILD
ELSE
(finally, specify BOTH, the trio card value and the wild card value as the values of cards to exclude)
SET valuesToExclude = [ CardValues.WILD, trioCardValue ]
END
}
(create the temporary kickers hand by excluding the specified values and returning the rest)
SET handOfKickers = CardMatchUtils.BuildHandExcludingValues(hand, valuesToExclude, numNonKickerCards)
(finally, sort the kickers hand in descending order, with wilds as the highest value)
handOfKickers = CardMatchUtils.SortCardsByDescendingValueHighWilds(handOfKickers)
RETURN handOfKickers
}
In the GetKickers
above, the info
object is the same one used in FindWinnerThreeKind
. It was the hand1Info
or hand2Info
object, depending on which hand you’re getting the kickers from.
There are three situations in GetKickers
that determine which values of cards to exclude as kickers.
The first is the simplest. It’s the trio card value used to form the three of a kind; they obviously can’t be the kickers. That means they will be excluded, leaving the remaining two cards as the kickers.
The second situation is that if the hand contains at least three wilds cards. These cards can assume the highest non-wild value, which is an ace. So three wilds would form a three of a kind, using an ace as the trio card value. So the wild card value would be excluded from the hand of kickers.
Finally, the hand doesn’t have three cards with the same value, but it does contain two wild cards. Those wilds will still assume the value of the highest non-wild card, forming the three of a kind. The trio card value is whatever value those wilds card assumed. In this situation, you want to exclude both values of both, the wild card, and the trio card value.
Note: When excluding cards, you’re always excluding exactly three cards (the number of non-kicker cards).
Comparing The Kickers
I hope you are still with me at this point. If you’re reading this far, I suppose it goes without asking 😉
When comparing which three-of-a-kind hand winds, if the trio values of both hands are the same, then you need to compare one or both of the kicker cards from both hands to determine the winner.
CompareKickers
CompareKickers = function(hand1, hand1Info, hand2, hand2Info) {
SET hand1Kickers = GetKickers(hand1, hand1Info)
SET hand2Kickers = GetKickers(hand2, hand2Info)
SET winningPlayerIndex = CardMatchUtils.CompareHands(hand1Kickers, hand2Kickers)
RETURN winningPlayerIndex
}
The CardMatchUtils.CompareHands
function is the same one we used here.
The CompareKickers
will return 0
if hand1 has the better kickers (and thus the better three-of-a-kind-hand), 1
if hand2 has the better kickers, or -1
if both hands are the same rank.
That’s it for this article. As we write more solutions, we can often re-use code from previous ones to help simply and speed up the process.
Compare Your Own Hands
You can use the controls below to build your own hands and compare threes of kinds. Have some fun playing around with it, and if you find an issue, please let me know!
Player 1
Player 2
Player 3
If you have any questions about the content in this article, go ahead and let me know at cartrell@gameplaycoder.com.
Thanks and take care,
– C. out.
Greater number will always win. This is the nature of card games, lower numbers have no place here.
Depends on the card game. In a straightforward comparison such as these, yes, higher ranked cards are better. But for something like blackjack, taking a hit and getting a low-ranking card can be the difference between beating the dealer or busting.
– C. out.