Continuing the series of comparing poker hands of the same rank, this article will show you how to write code to compare two straights to see which one wins.
In a previous article for poker hands, you saw how to check a hand to determine if a hand was a straight. A straight is a hand where the values of all five cards are in sequential order. Here’s an example of a straight:
Comparing Straight Hands
When both hands are a straight, it’s very easy to compare the hands. First, sort the cards in order of descending value, meaning from highest to lowest. Next, compare the first card (highest) card from each hand. The hand with the higher card is the hand with the higher-ranking flush. If both cards are equal, then both hands are of equal rank.
Have a look at this example, hand 2 has the higher-ranking flush, since a jack outranks an eight.
This works very well for card values two through ace. But what about dem pesky wild cards? 😼
Comparing Straight Hands With Wild Cards
Wildcards do complicate things a little, but it’s nothing we can’t handle. 😏
Note: You can’t compare straights with wild cards in them, so you have to substitute them for the correct values. Before, when all you were doing was determining if a hand is a straight or not, this was not necessary. However, before you can do a comparison, you need to have all the wilds gone.
When you have wildcards in a straight hand, you can do these three steps:
- Substitute card values for any values in between the highest and lowest card to fill out the straight. Use as many wilds as needed to fill out the gap between the highest and lowest card.
- If there are any wilds left over, and the highest card is not an ace, substitute one wild with a card value higher than the highest card.
- Repeat step 2 until you have replaced a wild card with an ace, or you run out of wilds.
- If there are any wilds left over, and the lowest card is not a two, substitute one wild with a card value lower than the lowest card.
- Repeat step 3 until you have replaced a wild card with a two, or you run out of wilds.
Some visual examples are always in order. 🙂
Example 1
Have a look at this hand:
Following step 1, you can substitute the two wilds with an eight and six, and you end up with:
Note: Remember, in a straight, as long as the hand contains at least two different suits, the suits don’t matter.
Example 2
Let’s try this hand:
Again, following step 1, we substitute wilds in between the highest and lowest values. Here, we’d use one wild and replace it with a nine, and we have:
We’ve one wild remaining, so we move on to step 2, and replace it with one higher than the highest card. So, you’d replace that wild with a queen, finally ending up with:
Example 3
Hey, lemme get another. Thanks, man. Good lookin’ out. 🤜🏾🤛🏾
Yep. (: If you’re holding these cards:
Step 1: There is no gap in between the queen and jack, so there’s nothing to do here.
Step 2: You can replace two cards higher then the queen, the king and ace. So you’d end up with this:
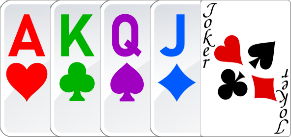
Step 3: With one wild left over, you replace it with one card lower than the lowest card, which currently is a jack. So, your final hand looks like:
Example 4
One final example. This one’s a bit of a smart-ass hand 😒
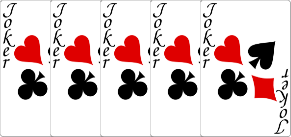
Here, you have no existing card values from which to establish a highest or lowest card. So to make the best possible straight, you can simply replace it with a hand that looks like the previous example’s solution:
However, there is a more programmatic way of doing this other than simply a brute force hard-code, which I’ll show you how.
Because there are no card values, you can establish a “fake” highest and lowest value of “ace + 1”. Since the highest and lowest values are the same, step 1 is skipped. Since your highest value is higher than an ace (somehow, lol), step 2 is skipped.
That leaves step 3, so you start replacing wilds with values lower than the lowest value, which is also “ace + 1”. So the first wild would substitute “ace + 1 – 1” = ace. Then the next substitution is one lower than the lowest value, ace, which is ace – 1 = king.
Setup
Note: The first stages of the setup can be found on the introduction page to comparing poker hands, here.
There aren’t any other specific preparations we need before we begin.
Writing The Code For Comparing Two Straights
When both hands are straights, the GetWinningPlayerId
function will eventually call the FindWinnerStraight
function that compares them to determine which one wins.
First, if either hand contains any wild cards, you’ll need to substitute them using the three steps mentioned above. Once all wilds have been substituted in both hands, you can compare them.
Let’s start with the FindWinnerStraight
function.
FindWinnerStraight
FindWinnerStraight = function(hand1, hand2) {
SET workingHand1 = CardMatchUtils.SortCardsByDescendingValue(hand1);
SET hand1Info to an empty object { }
CardMatchUtils.AreCardsStraight(workingHand1, hand1Info)
SET workingHand1 = WildsAssumeValues(workingHand1, hand1Info)
SET sortedHand2 = CardMatchUtils.SortCardsByDescendingValue(hand2);
SET hand2Info to an empty object { }
CardMatchUtils.AreCardsStraight(workingHand2, hand2Info)
SET workingHand2 = WildsAssumeValues(workingHand2, hand2Info)
SET winningPlayerIndex = CardMatchUtils.CompareHands(workingHand1, workingHand2)
RETURN winningPlayerIndex
}
In the psuedocode above, you may have noticed the AreCardsStraight
function from before, but it now has a new parameter that wasn’t there before: “info”.
We’ll need to make some adjustments to the AreCardsStraight
, because if wilds are in the hand, we want to know what values will be replacing these wilds (using the three steps above). We can store the values in the info.cardValues
array, same one we’ve used in previous hand comparing functions.
So, our new ‘AreCardsStraight’ function looks like this:
AreCardsStraight
AreCardsStraight = FUNCTION(sortedHand, info) {
SET numWilds to CardMatchUtils.CountCardsByValue(sortedHand, CardValues.wild)
SET cardValues to an empty array []
SET currentCardValue to 0
(these will be used later, but we want to set them up here)
SET highestCardValue to null
SET lowestCardValue to null
FOR EACH card in the sortedHand {
IF this card (the current loop card) is a wild card
IF (highestCardValue EQUALS null)
(this is the first card being checked)
SET highestCardValue to CardValues.ace + 1
END
EXIT FOR EACH LOOP
END
IF (this card is the first card being checked)
SET currentCardValue to the value of this card (card.value)
SET highestCardValue to currentCardValue
CONTINUE FOR LOOP again with next card (in other words, "continue" if you're familiar with for-loops)
END
SET valueDifference to currentCardValue - card.value
SET currentCardValue to card.value
IF valueDifference EQUALS 1
CONTINUE FOR LOOP again with next card (the condition for the straight is met so far)
END
IF valueDifference EQUALS 0
RETURN FALSE (this card has the same value as the previous card's stored in currentCardValue, the card from the previous loop iteration - this hand is not a straight)
END
SET lowestCardValue to currentCardValue
(check if there are enough wilds remaining to cover the difference in card values)
SET numWildsToUse to valueDifference - 1
IF numWilds GREATER THAN 0 AND numWilds IS GREATER THAN OR EQUALS numWildsToUse
(there are enough wilds, deduct that from the number of wilds available, and straight is still satisfied
SET numWilds to numWilds - numWildsToUse
(fill in the actial card values that will be replacing the wilds)
SET wildIndex to 0
WHILE wildIndex IS LESS THAN numWildsToUse
(repeat these steps as long as the WHILE condition is true)
SET substitutedCardValue to cardValue + wildIndex + 1
ADD substitutedCardValue into array cardValues
SET wildIndex to wildIndex + 1
END
ELSE
RETURN FALSE (there are not enough wilds to cover the range in value between the two cards - this hand is not a straight)
END
END
(straight is satisfied through all cards - success!)
(if there are any wilds remaining, try to assume values higher than the highest non-wild)
IF highestCardValue EQUALS null
SET highestCardValue to CardValues.ace
END
SET numWildsToUse to numWilds
WHILE (numWildsToUse IS GREATER THAN 0) {
SET cardValue to highestCardValue + (numWilds - numWildsToUse) + 1
IF (cardValue IS LESS THAN OR EQUALS CardValues.ace)
ADD cardValue into array cardValues
SET numWildsToUse to numWildsToUse - 1
ELSE
EXIT WHILE LOOP
END
END
(if there are any wilds remaining after assuming the higher values, try to assume values lower than the lowest non-wild)
IF (lowestCardValue EQUALS null
SET lowestCardValue to highestCardValue
END
SET offset to 0
WHILE offset IS LESS THAN numWildsToUse
SET cardValue to lowestCardValue - offset - 1
ADD cardValue into array cardValues
SET offset to offset + 1
END
IF info IS SPECIFIED
SET info.numWilds to numWilds
SET into.cardValues to array cardValues
END
RETURN TRUE
}
Wow! 😮 This is a little more than our original AreCardsStraight
function! But it now handles all three steps of replacing wilds with the correct card values. If you got nothing else from this, know that the AreCardsStraight
can now return to you those cardValues. 😉
Note that this function does not replace the cards in the hand itself. We’ll be doing that next; the main function of AreCardsStraight
is still to determine if a hand is straight, and you can specify an optional info
only if you need to know how many wilds are in the hand, and which card values would replace them to make a wild-less hand.
Replacing The Wild Cards With Card Values
Going back to the FindWinnerStraight
, you maye have noticed a new function you’ve never seen before, called WildsAssumeValues
. It accepts two parameters, a hand (an array or Card
objects), and an info object. What this function will do is return a new hand, with all the wilds replaced with the values specified in the info.cardValues
array.
Let’s have a look.
WildsAssumeValues
WildsAssumeValues = function(hand, info) {
SET index to 0
WHILE index IS LESS THAN number of cards in the hand (for example, "hand.length")
SET card = hand[index] (get the nth card in hand)
IF the card is a wild (card.value EQUALS CardValues.wild)
SET the card value to the last value in the info.cardValues array
REMOVE the last card value from the info.cardValues array (info.cardValues.pop())
END
SET index = index + 1
END
SET sortedHand to CardMatchUtils.SortCardsByDescendingValue(hand)
RETURN sortedHand
}
All the WildsAssumeValues
does is go through the hand, replacing any wilds it finds with a value from the info.cardValues
array. Then, it removes that value from the array to make sure it’s not used again. Finally, it creates a new hand and sorts the cards by card value in descending order, and returns that new hand. This new hand is what we’ll use to compare straights with.
Back in the FindWinnerStraight
function, at this point, you’ll now have two hands with any wilds replaced with actual card values, and you can finally compare both hands using the CardMatchUtils.CompareHands
function.
Remember, CardMatchUtils.CompareHands
will return 1
if the first hand wins, -1
if the second hand winds, or 0
if both hands are tied.
That’s it for comparing two straight hands! Now, if you like, you can play with creating your own hands using the controls below. Enjoy! 😎
Compare Your Own Hands
Player 1
Player 2
Player 3
If you have any questions about anything in this article, please let me know at cartrell@gameplaycoder.com.
Thanks, take care, and until next time,
– C. out.
I’d actually love to see a real life game where somebody gets 5 Jokers. Of course they’ll know they’re being played right then and there.
All the other players might think they’re getting played. How’d this bloke get 5 jokers!? Then the dealer gets looked at.
– C. out.